One of the essential skills in todays e-commerce developers toolbox is JavaScript. JavaScript, originally developed by Netscape and released as LiveWire, has undergone a few transformations since its inception, even finding its way to the server side of e- commerce applications. Probably more often than not, however, its still used primarily on the client or, more specifically, with the browser. Ill show you how to use it for one of the more common tasks in e-commerce development: form validation.
A Short History Lesson
JavaScript is a common term to most, but some may still be wondering how JScript or even ECMAScript fit in the scheme of things. By now, you should be painfully aware of the battles between Microsoft and Netscape. Everybody knows about the browser wars, but a lesser-known battle within that war is over scripting. Shortly after Netscape developed JavaScript, it was turned over to the European Computer Manufacturers Association (ECMA), an international standards organization. ECMA issued its standard, commonly known as ECMA-262, which has the title of ECMAScript language specification. Both JScript and JavaScript have arisen from this specification. ECMA-262 attempts to solve the matter of cross-browser compatibility, but this being the real world, there are obstacles to overcome. Microsofts standard practice of extending technologies means that JScript is ECMA-262-compliant and then some, adding a few more features here and there. Additionally, Netscape runs a little behind when it comes to keeping Navigator in pace with ECMA-262, although its JavaScript Version 1.3 claims full compliance.
JavaScript can reside in a source file external to the HTML page using the source, or it can be embedded directly into the HTML. When a JavaScript-enabled browser comes across a Web page that has JavaScript in it, the browser activates its scripting engine and begins to execute, or interpret, the JavaScript code. Without poring through the details of the ECMA standard, its hard to say which browser complies with the standard. Without going into too much detail about the ECMA-262 document (which can be downloaded at www.ecma.ch), Ill leave it up to you to read the specification and see how it leaves the two scripting languages plenty of wiggle room, especially concerning the event model. Nevertheless, both scripting languages claim that they are in full compliance with ECMA- 262; however, there are still some instances where certain object properties and methods
work in either Navigator or Internet Explorer but not both. That is to say, cross-browser JavaScript is still the burden of the developer.
Fortunately, there is a way within JavaScript to detect the browser and version by which the developer will want to condition the processing of the script. For example, the following code snippet will detect your browsers name and version. It works for all browsers despite using the navigator object.
var browser = navigator.appName;
var version = navigator.appVersion;
JavaScript for Client-side Validation
Among the many things you can use JavaScript for is to validate data that a user inputs into a form before that data gets sent across the wire to whatever you have waiting for it on the server. This use is probably the most common use of JavaScript in Web application programming. Your server-side component may be a Java servlet, an Active Server Page (ASP), or a CGI script. Whatever the component is, you dont want it to choke, gasp, and wheeze by sending it data that it isnt prepared to handle. JavaScript is an excellent choice to handle this task because all the validation is done on the client, i.e., the browser. Validation done through the browser gives far greater performance than having to send that form data to the server, having some sort of server-side data validation, and then having the server send back to the browser any errors that may have occurred. Sometimes this situation is unavoidable, and Ill talk about that later.
There are several aspects to form validation, and Ill cover them one-by-one. Ill start out by discussing a little of whats known as the event model. Every browser has its event model, which means that each browser has a way to let JavaScript know what event is taking place. For example, when a user presses a key on the keyboard, the onKeyPress event takes place, and if your JavaScript code does some processing on the onKeyPress event, it will be executed at that time. A major event for form validation is the onSubmit event, which is what normally kicks off form validation. The onSubmit event typically happens when a user presses an HTML button, created by the tag, that has Submit as the value for the tags Type parameter.
Common tasks involved with form validation are checking for missing values in required fields, checking the format of the data, providing the user with drop-down lists of valid data to enforce validity, or prompting the user for valid data á la the F4 key in OS/400.
Checking for missing values in required fields is relatively easy. Assuming a form called myForm was submitted as a form to be validated by a script, the way to check if FieldA contained any value at all is as follows:
if(myForm.FieldA.length == 0)
.. processing for missing data
Once a field that has data in it is passed to your validation script, youll probably want to make sure its in the format you want before handing it off to the server. The best example of this is validating dates. Of course, youll want to make sure that your dates are valid, that theyre in the correct month-day-year sequence, and that they have a valid date separator. Thats actually a lot to check for most developers, but since many of you have plenty of experience in RPG III, Im sure youre not scared to perform this basic but essential task.
Figure 1 is a simple HTML form with a collection of required fields to be validated. Ive included a downloadable example of Figure 1 at www.midrangecomputing. com/mc. Look at the drop-down list of states. Youll notice that the first value in the list is a text value: Select a state. By default, it is the value that is selected when the form first loads. You want to make sure that a user selects a value in the drop-down list other than the first
value, which is commonly a request to select a value, as in this example. To validate this field, youll use the following code:
var state = form.state.selectedIndex;
. . .
if (state == 0)
{
errors[count++] = State error: +
Must select a state. ;
}
The variable called form is the object that is passed to the validation script. A property of any form is a field name, in this case, State. Because State is a drop-down list created by the HTML tag
Is That a Regular Expression?
A good way to validate the format of values entered into fields is by using what is known as a regular expression. The fields of zip code, phone number, birthday, and social security number are shown to give you a little introduction. Regular expressions are rooted in research that was done on the human nervous system back in the 1950s, and later, when UNIX was invented, they were called upon to provide the foundation for search algorithms. Regular expressions are quite common in UNIX environments and have shown up in JavaScript as well, which is a good thing, because without regular expression support, validating fields would be a more involved programming task. There is no better way to kick off a discussion about regular expressions than to show you what one looks like.
For a zip code in the United States, there are really only two formats. A five-digit number or a five-digit number followed by a four-digit number separated by a dash. The following formats are two possible ways to show the two zip code formats as regular expressions:
/^(d{5})$/
/^(d{5})(-)(d{4})$/
As you can see, the format of regular expressions is not exactly intuitive. Ill break apart the second regular expression to look at some of the syntax. In JavaScript, regular expressions are contained within slash (/) characters as this one is. The caret (^) character signals the beginning of an input line, meaning a line that will be matched up against the regular expression. You can see that there are three groups of characters or patterns enclosed in parentheses. This is just a matter of programming style. Ive gotten into the habit of breaking apart the regular expressions into these patterns because when they are matched against a string, I can get at the values of those patterns in an array thats created when the matching takes place. Ill give you more on that later. The first and third patterns are basically the same. The backward slash () character indicates that some kind of special character is next. In this case, its the letter d character, which is used to match a digit 0 to
9. The values placed in enclosing brackets, 5 in the first pattern and 4 in the third pattern, tell the expression to match that many times for the value before them. In this case, it was the special character d that means for the first pattern, check for five digits, and for the third pattern, check for four digits. The dollar sign ($) character signals the end of the
input line. At the end of this article, Ive referenced a couple of links where you can find out more about regular expressions.
Assuming you are comfortable with regular expression syntax, how do you use the things? Here is some code from the example to validate the birthday field:
var datePat = /^(d{2})(/)(d{2})2(d{2}|d{4})$/;
var dateMatchArray = bday.match(datePat);
Ive declared a variable called datePat, which is essentially the regular expression. I then declare an array that is populated by matching the birthday field against the regular expression. Take a second look at the snippet. How is the array populated? Whats in the array? The array is populated as a result of calling the match() method: The array is populated with any and all matches for the entire string being matched against the regular expression and the values of the patterns mentioned earlier. In this example, a valid date will be the first value in the array followed by the values of the month, date separator, day, and year. A date is a good example of not only validating an entire string, but also breaking apart values into the patterns of the regular expression. That way, the month can be validated as well as the day to see if the combination of the two is valid. The example takes care of that scenario as well.
Handling Errors
What happens when the form has errors in it? I mentioned how errors are sent into an error array to be displayed for the user. I suppose that this topic falls under the category of programming preference and style, but Ive always thought it to be good practice for a form to collect as much error information as possible and display it at once rather than catching an error, showing an error dialog box, and iterating through that process until all the errors have been caught and corrected. Figure 2 (page 95) shows what Im talking about. The error shows that Ive forgotten to select a state from the drop-down list and that Ive entered incorrect values for the zip code, phone number, and birthday fields. Rather than catching the first error (not selecting a state) and displaying that error for the user to correct, I populate an error array that is in fact a String array and then cycle through the array at the end of the script, formatting the elements of the error array into one String object to be displayed in the JavaScript alert window.
Detecting JavaScript Support
Now, some of you may be more familiar with JavaScript than others, and, while reading this article, you may think, Yeah, but what if the user disables JavaScript in the browser? Its true, all of your good form-validating intentions and whiz-bang JavaScript could be for naught if the browser displaying your page has no JavaScript capabilities or has those capabilities disabled. What then? In this situation, your users will be able to submit just about any value they wanted if form validation depended solely on JavaScript. You could validate all the data on the server but that would defeat JavaScript validation altogether and would be a waste of server resources. JavaScript forums and newsgroups are littered with a common question JavaScript newcomers often ask: How can I write a script that will detect if JavaScript is disabled or not? The answer, of course, is you cant, because if JavaScript is disabled, no detection script will be able to run. A little trick that I use in this particular example is to put a hidden field inside the form called validation. If you look inside the examples HTML, youll see it has a value of no. In the script, if there are no errors, I set the fields value to yes. On the server, youd have to have a component check the value of that field, and if it is yes, then JavaScript did its job in checking the field values before they were passed to the server. If its no, then JavaScript couldnt change the value from its original state, indicating that JavaScript was disabled on the browser that sent the form. In this case, either a server-side component would be responsible for data
validation, or you could send back a standard reply to the users browser informing him that JavaScript is required to use the site.
Form validation is a great and sometimes necessary way to become acquainted with JavaScript. Also, as an AS/400 developer, youre already acutely aware of how important form validation is in creating business applications. Combine this client-side technology with your already mature server-side knowledge so that your browser-based applications are as efficient and well-rounded as possible.
REFERENCES AND RELATED MATERIAL
Client-side JavaScript Reference (online reference manual available at http://developer. netscape.com/docs/manuals/js/client/jsref/index.htm)
ECMA home page: www.ecma.ch
Microsoft Windows Script Technologies: msdn.microsoft.com/scripting/
Figure 1: This form will be validated with JavaScript.
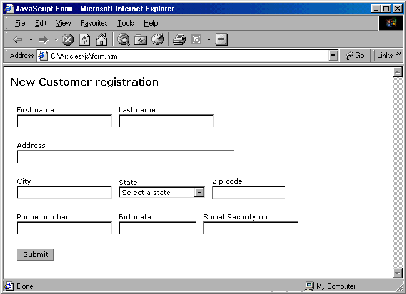
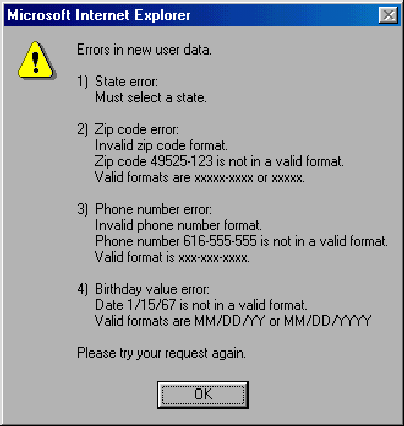
Figure 2: Display as many error messages as you can in a single dialog box.
LATEST COMMENTS
MC Press Online