Pretty much everyone in the IT industry uses a PC—either at work, at home, or both. At times, you’ve probably used your PC to get on the Internet or to connect to your AS/400 using TCP/IP. You’ve also most likely used FTP to transfer files from one location to another. So, you know that FTP is a pretty handy little weapon to have in your arsenal. It’s fast, it’s easy to use, and, best of all, it’s free. Many of you routinely use FTP on your AS/400 to transfer files between AS/400s (hereafter referred to as iSeries). And FTP works really well for that purpose.
Introducing the FTP Classes
One area that doesn’t get written about too much, however, is using FTP to transfer files between an iSeries and a PC. Professionals in the iSeries media tend to gloss over this necessary aspect of FTP. The authors offer one or two tips on how to use FTP with a PC, from a DOS window, or how to create a batch FTP file transfer script, and rarely go any farther. Well, the truth is that until the advent of the latest version of the AS/400 Java Toolbox, called JTOpen, there just wasn’t an easy way to use FTP with your iSeries from anything but a DOS window. All that has changed with the inclusion of the FTP classes in the AS/400 Java Toolbox. In this article, I’ll tell you about the FTP classes and what you can do with them. Then I’ll show you a little Java utility I wrote that uses these classes to get files from an iSeries and stores these files on your PC.
IBM created the Java FTP classes and included them in the com.ibm.as400.access package in the Java Toolkit. Using these classes, you can perform pretty much any FTP operation in much the same way that you would if you were running these commands from a DOS command line. The nice thing about these classes is that you can programmatically run FTP commands rather than force your users to hop out of their PC applications and open a DOS session to execute the commands. This feature opens up a whole world of new functionality, which you can build into your Java applets or applications. Now you can design a Java app to run in your users’ browsers, for example, that lets users integrate the power of FTP into their daily routines.
One Way You Might Use It
For example, imagine that you have a user performing inventory item maintenance on the iSeries. You’ve designed a JavaServer Page (JSP) to serve the item information to the
user’s Web browser, which he uses as his main interface to the iSeries. Now assume even further that this user works closely with another user who is on another iSeries in another state. Both iSeries machines at both locations are connected to a TCP/IP network, but neither is connected to the World Wide Web. Imagine that your user needs to get a data file (an iSeries physical file) containing item pricing information to the off-site user. There are many ways you could get this file to that off-site user; perhaps the easiest method would be to send it to the off-site user as an iSeries save file (*SAVF). Using the *SAVF format, you could ensure that the data is restored on the off-site user’s system exactly as the data was saved on the local system.
By using the Java FTP classes, and, in this specific case, the Java AS400FTP subclass, you can easily integrate the FTP functionality you need on both iSeries systems. Here’s how this scenario might play out:
• From the local browser, the user identifies the iSeries physical file he wants to save and distribute. Or, perhaps, you might always use the same file name and select the file for him automatically.
• Still in the local browser, the user issues an iSeries command to save the physical file to an iSeries *SAVF.
• The browser applet on the local system runs the AS400FTP subclass. This subclass is intelligent enough to know that for *SAVF files, the local system needs to perform a binary transfer for the download to avoid data corruption.
• The local browser applet opens an email window and attaches the downloaded *SAVF to the email as an attachment.
• The local user types in a descriptive message and sends the email to the off-site user.
• The off-site user retrieves the email either manually or through the Java mail classes. Using the former method, the off-site user would save the attachment to his local hard drive. Using the latter, your application might process the attachment in place.
• From his browser applet, the off-site user clicks on a button you provided in the applet’s GUI, which uses the Java AS400FTP subclass to FTP the *SAVF to his local iSeries, where the file can then be restored via a command issued from the browser.
What’s Included
That’s just one possible scenario for using the FTP classes. You can probably think of other ways to use these classes. But, before you can start using the classes, you need to know what’s available. Included in the Java FTP classes are the following primary methods:
• Connect—Connect to an iSeries FTP server
• Send—Send commands to the FTP server
• Get—Retrieve objects from the FTP server
• Put—Send data/objects to the FTP server
Of course, there are several other methods such as List, cd, setDataTransferType, and others that you’ll probably want to use with the FTP classes. In addition to these methods, there is also the AS400FTP subclass, which I mentioned earlier. This subclass is a derivative of the FTP class, which has a lot of the iSeries requirements for FTP already built into it. For example, this subclass knows that an iSeries *SAVF must exist prior to
sending a *SAVF to the host, so this subclass will automatically create that *SAVF if it doesn’t exist. This subclass also has a few other unique features, but the *SAVF feature is probably the most useful.
Once you start looking into and using the AS/400 toolkit’s FTP classes, you’re going to discover that they are very easy to use. In fact, the following code snippet illustrates everything you need to do to use these classes to retrieve a file from an iSeries using the AS400FTP subclass. As you can see, it doesn’t take much to start using these very powerful classes.
import com.ibm.as400.access.FTP.*;
import java.io.*;
public class TestFTP {
public static void main(String[] argv) {
AS400 system = new AS400();
AS400FTP ftp = new AS400FTP(system);
try {
ftp.get(“//home//yourfile.txt”, “C: ewfile.txt”);
ftp.disconnect();
System.exit(0); }
catch(IOException ie) {}
}}
A Look at the Utility
I’ve written a very simple Java application to demonstrate how you might use the FTP classes. Figure 1 shows what the application looks like. As you can see, it’s very primitive. The application has a few buttons on it, a list window, and a text field. When you click on the Add File button, a Java IFSFileDialog window will open to display a list of files and directories on your iSeries. Select a file to be downloaded to your PC, and it will be added to the list window. You can continue to select files until you get as many as you want or need. Next, you click on the Select Destination button to open a window that allows you to select the directory you want to FTP the files to. Finally, click on the Start FTP button to begin the FTP process. The program will connect to your iSeries FTP server, get the files in the list from the AS/400 Integrated File System (AS/400 IFS), and then place the files in the local PC directory you chose. When the program is done, the list will be cleared and the text field at the bottom of the GUI will display the message “FTP Complete.” This example can be run as an application or as an applet. Be warned, however, that the JFileChooser I use in the code to allow the user to select a destination directory does not run in all browsers. Therefore, if you choose to modify and use this code for your own purposes, you may want to come up with another method of choosing a destination directory that will work in any browser. This utility is for demonstration purposes only. You’ll probably want to examine the techniques in the code to see how I used the FTP classes and then design your own applications.
The code and a working example of this utility can be downloaded in a zip file from www.midrangecomputing.com/mc. When you download the file to your PC, unzip it to a directory of your choice and double-click the SETUP.exe program in that directory. A directory named Midrange Computing will be created under the Program Files directory on your PC. Inside the Midrange Computing directory will be another directory named FTPApplet, and that’s where you’ll find the source code for this example. Also, an entry will be placed on your Programs Menu called Midrange Computing Software. In that menu will be an item called FTPApplet, and in that item will be another item called FTPApplet.bat. You can run this example by selecting the FTPApplet.bat menu item. If you want to try this as an applet, open the FTPApplet.html file in the source directory. You may also need the Java Runtime Environment, if you don’t already have one on your PC
(this can be downloaded for free from Sun Microsystems at www.javasoft.com/ products/).
Piece of Cake!
For more detailed information on how to use the FTP classes, look in the AS/400 Java Toolkit documentation, which you can download from IBM at www.as400.ibm.com/ toolbox. The FTP classes are described under the ACCESS category. Once you start looking at the documentation and begin using these classes, you’ll find that they are so easy to use that you’ll probably integrate them into your software the very first day!
Figure 1: This primitive GUI packs all the power of FTP inside!
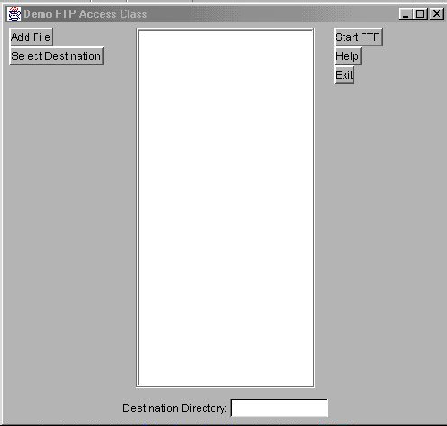
LATEST COMMENTS
MC Press Online